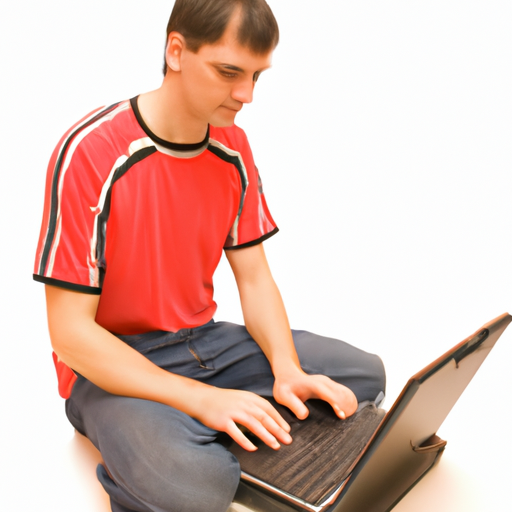
Mastering User Input in C: A Beginner’s Guide
User input is a critical component of any programming language, and C is no exception. Whether you are designing a simple command-line tool or a full-fledged software application, understanding how to handle user input in C is essential. In this beginner’s guide, we will explore the various techniques and best practices for mastering user input in C.
Understanding User Input in C
Before we delve into the specifics of handling user input in C, it’s important to understand the basics of user input. In C, user input is typically received through standard input, which is generally associated with the keyboard. Standard input is represented by the FILE pointer stdin, and you can use functions like scanf and fgets to read input from the user.
The scanf function is commonly used for reading formatted input, while the fgets function is used for reading a line of text from the user. Both functions have their own advantages and disadvantages, and we will explore them in more detail later in this guide.
In addition to standard input, C also provides standard output and standard error, represented by the FILE pointers stdout and stderr, respectively. These are used for displaying output and error messages to the user.
Handling User Input Using scanf
As mentioned earlier, the scanf function is commonly used for reading formatted input from the user. The basic syntax of the scanf function is as follows:
“`c
int scanf(const char *format, …);
“`
The format parameter specifies the format of the input that the function should expect. For example, if you want to read an integer from the user, you can use the “%d” format specifier. Similarly, if you want to read a floating-point number, you can use the “%f” format specifier.
Here is an example of how to use the scanf function to read an integer from the user:
“`c
int num;
printf(“Enter a number: “);
scanf(“%d”, &num);
printf(“You entered: %d\n”, num);
“`
In this example, we first prompt the user to enter a number using the printf function. We then use the scanf function to read the input and store it in the variable num. Finally, we use the printf function again to display the input back to the user.
While the scanf function is quite versatile, it does have some limitations. One of the main drawbacks of scanf is that it does not handle newline characters properly, which can lead to unexpected behavior when reading input from the user. Additionally, scanf does not provide a way to limit the length of the input, which can make it vulnerable to buffer overflow attacks.
Handling User Input Using fgets
To overcome the limitations of the scanf function, you can use the fgets function to read a line of text from the user. The basic syntax of the fgets function is as follows:
“`c
char *fgets(char *str, int n, FILE *stream);
“`
The str parameter specifies the buffer where the input should be stored, and the n parameter specifies the maximum number of characters to read. The stream parameter should be set to stdin to read input from the user.
Here is an example of how to use the fgets function to read a line of text from the user:
“`c
char input[100];
printf(“Enter some text: “);
fgets(input, sizeof(input), stdin);
printf(“You entered: %s\n”, input);
“`
In this example, we use the fgets function to read a line of text from the user and store it in the input buffer. We then use the printf function to display the input back to the user.
The fgets function is a safer alternative to scanf, as it handles newline characters properly and allows you to limit the length of the input. However, it does require you to manually parse the input and convert it to the desired data type, which can be cumbersome for complex input formats.
Validating User Input
When handling user input in C, it’s important to validate the input to ensure that it meets your application’s requirements. For example, if you are expecting the user to enter an integer, you should check that the input is indeed an integer before using it in your program.
One way to validate user input is to use the scanf function’s return value. The scanf function returns the number of items successfully read, so you can use this value to determine if the input is valid. For example:
“`c
int num;
printf(“Enter a number: “);
if (scanf(“%d”, &num) == 1) {
printf(“You entered: %d\n”, num);
} else {
printf(“Invalid input\n”);
}
“`
In this example, we use the return value of scanf to check if the input is a valid integer. If the input is valid, we display it to the user; otherwise, we display an error message.
Another way to validate user input is to use the strtol function to convert the input to a long integer and check for errors. The strtol function has the following syntax:
“`c
long int strtol(const char *str, char **endptr, int base);
“`
The str parameter specifies the input string, and the base parameter specifies the number base to use for the conversion (e.g., 10 for decimal). The endptr parameter is used to store the end of the conversion, which can be useful for error checking.
Here is an example of how to use the strtol function to validate user input:
“`c
char input[100];
char *endptr;
long int num;
printf(“Enter a number: “);
fgets(input, sizeof(input), stdin);
num = strtol(input, &endptr, 10);
if (input != endptr && *endptr == ‘\n’) {
printf(“You entered: %ld\n”, num);
} else {
printf(“Invalid input\n”);
}
“`
In this example, we use the strtol function to convert the input to a long integer and check for errors. If the input is valid, we display it to the user; otherwise, we display an error message.
Conclusion
Handling user input in C is a fundamental skill that every programmer should master. Whether you are designing a simple command-line tool or a complex software application, knowing how to properly handle user input is essential for creating robust and user-friendly programs.
In this beginner’s guide, we explored the various techniques and best practices for mastering user input in C. We discussed the scanf and fgets functions for reading input from the user, as well as the importance of validating user input to ensure its correctness. Armed with this knowledge, you can confidently handle user input in your C programs and create applications that are both reliable and user-friendly.