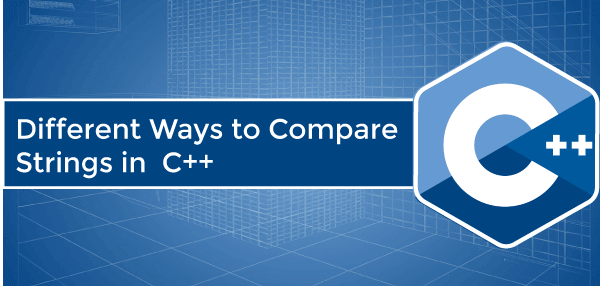
String comparison is a fundamental operation in programming, and mastering it can greatly improve the quality, reliability, and efficiency of your code. This is particularly true in the C programming language, where strings are a core component of many applications. In this beginner’s guide, we will explore the art of string comparison in C, from the basic principles to advanced techniques that will help you write better code.
Before we dive into the specifics of string comparison in C, let’s first define what a string is. In C, a string is a sequence of characters terminated by a null character (‘\0’). This means that any array of characters that ends with the null character is considered a string in C. For example, the string “hello” is represented as an array of characters {‘h’, ‘e’, ‘l’, ‘l’, ‘o’, ‘\0’}.
Now that we understand what a string is in C, let’s move on to the basic principles of string comparison. In C, string comparison is typically performed using the strcmp() function, which is defined in the header file. The strcmp() function takes two strings as arguments and returns an integer value based on the lexicographical comparison of the strings. If the first string is less than the second string, strcmp() returns a negative value. If the first string is greater than the second string, it returns a positive value. If the two strings are equal, it returns 0.
Here’s an example of how the strcmp() function is used to compare two strings in C:
“`c
#include
#include
int main() {
char str1[] = “abc”;
char str2[] = “def”;
int result = strcmp(str1, str2);
if (result < 0) {
printf(“str1 is less than str2\n”);
} else if (result > 0) {
printf(“str1 is greater than str2\n”);
} else {
printf(“str1 is equal to str2\n”);
}
return 0;
}
“`
In the example above, we have two strings “abc” and “def”. We use the strcmp() function to compare them, and based on the result, we print a message indicating whether the first string is less than, greater than, or equal to the second string.
While the strcmp() function is a simple and effective way to compare strings in C, there are cases where a more customized comparison is needed. For example, you may need to compare strings in a case-insensitive manner, or you may need to compare strings with a specific locale.
To address these cases, C provides the strcoll() function, which is defined in the header file. The strcoll() function is similar to strcmp(), but it uses the current locale to perform the comparison. This means that it takes into account the language-specific sorting rules and conventions when comparing strings. If you need to compare strings in a case-insensitive manner, you can use the strcasecmp() or strncasecmp() functions, which are also defined in the header file.
In addition to these standard string comparison functions, C also provides ways to perform advanced comparison operations on strings. For example, you can use the memcmp() function to compare a specific number of bytes in two strings, or the strxfrm() function to transform a string according to the current locale and then compare it using strcmp().
Another important consideration when comparing strings in C is handling memory allocation and freeing. When working with strings, it’s important to ensure that memory is allocated and freed appropriately to avoid memory leaks and undefined behavior. This is particularly important when comparing dynamically allocated strings, as failing to properly manage memory can lead to serious bugs and security vulnerabilities.
To illustrate the importance of memory management in string comparison, consider the following example:
“`c
#include
#include
#include
int main() {
char *str1 = malloc(10 * sizeof(char));
char *str2 = malloc(10 * sizeof(char));
strcpy(str1, “abc”);
strcpy(str2, “def”);
int result = strcmp(str1, str2);
if (result < 0) {
printf(“str1 is less than str2\n”);
} else if (result > 0) {
printf(“str1 is greater than str2\n”);
} else {
printf(“str1 is equal to str2\n”);
}
free(str1);
free(str2);
return 0;
}
“`
In this example, we dynamically allocate memory for two strings using the malloc() function, and then compare them using the strcmp() function. After the comparison is done, we free the allocated memory using the free() function to avoid memory leaks.
In addition to memory management, it’s also important to handle edge cases and unexpected input when comparing strings in C. For example, when comparing strings of different lengths, it’s important to ensure that the comparison is performed safely and without causing buffer overflows or underflows. Similarly, when comparing user input or external data, it’s important to validate and sanitize the input to prevent security vulnerabilities and unexpected behavior.
To summarize, mastering the art of string comparison in C is essential for writing reliable and efficient code. By understanding the basic principles of string comparison, using the standard comparison functions provided by C, and paying attention to memory management, edge cases, and unexpected input, you can develop a deep understanding of string comparison and write better code.
In the next section, we will explore some advanced techniques and best practices for string comparison in C, including handling different locales, case-insensitive comparison, and comparing dynamically allocated strings.
One advanced technique for string comparison in C is handling different locales. In multilingual applications, it’s important to take into account the language-specific sorting rules and conventions when comparing strings. C provides the strcoll() function for this purpose, which uses the current locale to perform the comparison. The strcoll() function is particularly useful when comparing strings in different languages or when sorting data based on locale-specific rules.
Another common requirement when comparing strings in C is performing case-insensitive comparison. C provides the strcasecmp() and strncasecmp() functions for this purpose, which compare strings in a case-insensitive manner. These functions are particularly useful when working with user input or external data, as they allow you to compare strings without considering the case of the characters.
In addition to locale-specific and case-insensitive comparison, C provides ways to compare specific portions of strings using the memcmp() function. The memcmp() function compares a specific number of bytes in two strings, allowing you to perform byte-level comparison operations. This can be particularly useful when comparing binary data or when performing low-level string manipulation.
Finally, C provides the strxfrm() function for transforming a string according to the current locale and then comparing it using strcmp(). The strxfrm() function is useful when you need to compare strings based on the transformed form, such as when performing locale-aware sorting operations.
In conclusion, mastering the art of string comparison in C is essential for writing reliable, efficient, and secure code. By understanding the basic principles of string comparison, using the standard comparison functions provided by C, and applying advanced techniques and best practices, you can develop a deep understanding of string comparison and elevate your programming skills.
In this beginner’s guide, we’ve covered the basics of string comparison in C, from the fundamental principles to advanced techniques that will help you write better code. Whether you’re writing a simple utility or a complex application, understanding how to compare strings effectively in C is a crucial skill that can greatly enhance the quality and reliability of your code. By following the best practices we’ve discussed and exploring the advanced techniques available in C, you’ll be well on your way to mastering the art of string comparison.